7. Assignment VII: Deep Learning#
7.1. Question 1#
Use the dataset, DEMO_DATA/chinese_name_gender.txt
and create a Chinese name gender classifier using the deep learning method. You need to include a few important considerations in the creation of the deep learning classifer.
Please consult the lecture notes and experiment with different architectures of neural networks. In particular, please try combinations of the following types of network layers:
dense layer
embedding layer
RNN layer
bidirectional layer
Please include regularizations and dropbouts to avoid the issue of overfitting.
Please demonstrate how you find the optimal hyperparameters for the neural network using
keras-tuner
.Please perform post-hoc analyses on a few cases using
LIME
for more interpretive results.
Show code cell source
plot_model(model1, show_shapes=True)
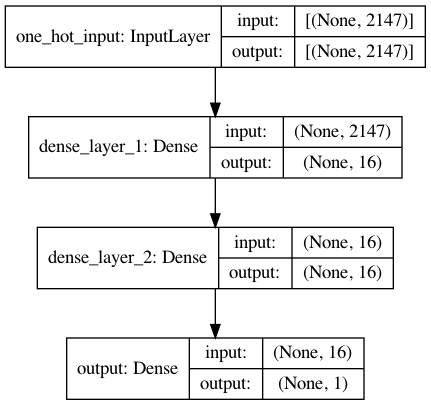
Show code cell source
plot_model(model2, show_shapes=True)
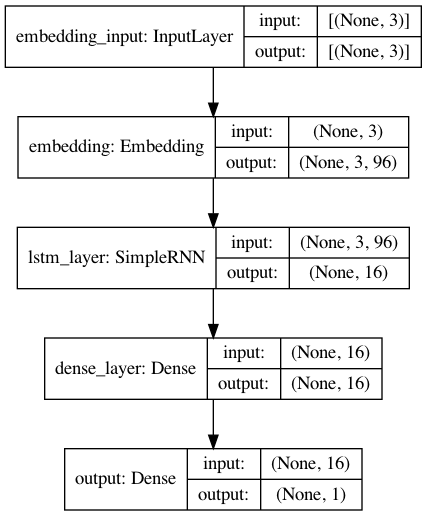
Show code cell source
plot_model(model3)
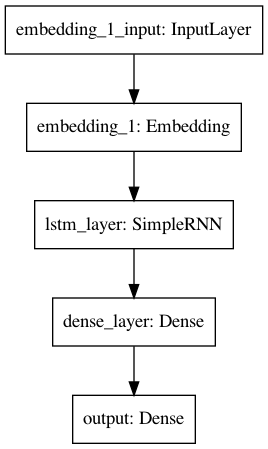
plot_model(model4)
Show code cell output
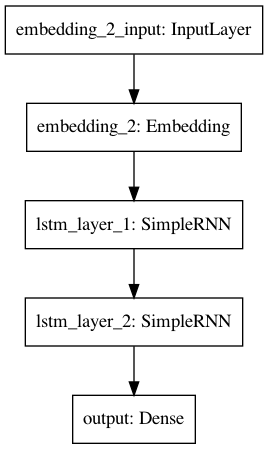
Show code cell source
plot_model(model5)
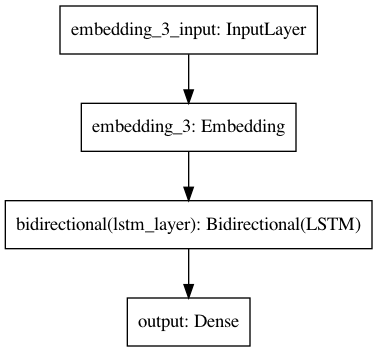
from lime.lime_text import LimeTextExplainer
explainer = LimeTextExplainer(class_names=['Male'], char_level=True)
Show code cell source
exp = explainer.explain_instance(
X_test_texts[text_id], model_predict_pipeline, num_features=100, top_labels=1)
exp.show_in_notebook(text=True)
Show code cell source
exp = explainer.explain_instance(
'陳宥欣', model_predict_pipeline, num_features=100, top_labels=1)
exp.show_in_notebook(text=True)
Show code cell source
exp = explainer.explain_instance(
'李安芬', model_predict_pipeline, num_features=2, top_labels=1)
exp.show_in_notebook(text=True)
Show code cell source
exp = explainer.explain_instance(
'林月名', model_predict_pipeline, num_features=2, top_labels=1)
exp.show_in_notebook(text=True)
Show code cell source
exp = explainer.explain_instance(
'蔡英文', model_predict_pipeline, num_features=2, top_labels=1)
exp.show_in_notebook(text=True)